Using DNS to save and read my cars data worldwide
October 26, 2024
When I wrote the simple code to stop loading my cars battery at 80%, I was looking for a way to save relevant car data to use it for further purposes. Relevant data was the cars battery state in percent, the charging target (80% in my case) and the mileage left on battery. In order to access this data from different devices worldwide I decided to store it in a DNS record.
Get a free DNS record
I created a free DNS zone at dynv6.com. Next step was to create a security token to update my DNS entry via API and then append a new line to my python code.
bimmerhtml = requests.get(“https://ipv4.dynv6.com/api/update?token=<your token goes here>&zone=<your DNS zone goes here>&ipv4=” + percent + “.” + target + “.” + range + “.0”)
In order to make HTTP requests work in the python script another library is neccessary to add in the head of the script.
import requests
That’s all and now you are able to read this data from other devices really simple.
An iPhone widget
My first use case was to display mileage left and battery state on my iPhones home screen.
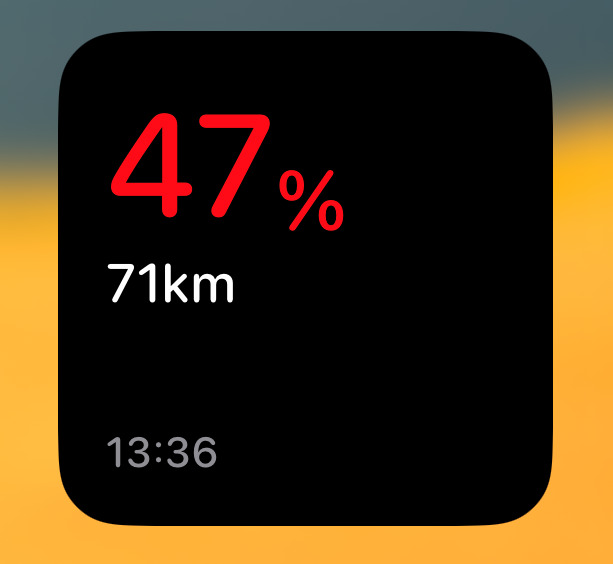
Within the scriptable app I wrote a small script to accomplish that:
//timestamp
let date = new Date();
let hours = date.getHours();
let minutes = date.getMinutes() < 10 ? “0” + date.getMinutes() : date.getMinutes();
let datum = hours + “:” + minutes;
url = “https://dns.google/resolve?name=<your DNS zone goes here>”;
req = new Request(url);
let answer = await req.loadJSON();
let dns = answer.Answer[0].data;
let octets = dns.split(“.”, 4);
percent = octets[0];
target = octets[1];
range = octets[2];
let widget = new ListWidget();
widget.backgroundColor = Color.black();
let stack = widget.addStack();
stack.bottomAlignContent();
let wpercent = stack.addText(percent);
wpercent.font = Font.mediumRoundedSystemFont(48)
wpercent.textColor = Color.red();
let wpercentsign = stack.addText(“%”);
wpercentsign.font = Font.mediumRoundedSystemFont(28)
wpercentsign.textColor = Color.red();
let wRange = widget.addText(range+”km”);
wRange.font = Font.mediumRoundedSystemFont(18)
wRange.textColor = Color.white();
wRange.leftAlignText();
widget.addSpacer();
let wDate = widget.addText(datum);
wDate.font = Font.mediumRoundedSystemFont(14)
wDate.textColor = Color.gray();
wDate.leftAlignText();
Script.setWidget(widget);
Script.complete();
App.close();
Have fun.